Learning Resources
Streams and Bytes Data
Byte Streams
Programs use byte streams to perform input and output of 8-bit bytes. All byte stream classes are descended from InputStream
and OutputStream
.
There are many byte stream classes. To demonstrate how byte streams work, we'll focus on the file I/O byte streams, FileInputStream
and FileOutputStream
. Other kinds of byte streams are used in much the same way; they differ mainly in the way they are constructed.
Using Byte Streams
We'll explore FileInputStream
and FileOutputStream
by examining an example program named CopyBytes
, which uses byte streams to copy xanadu.txt
, one byte at a time.
import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; public class CopyBytes { public static void main(String[] args) throws IOException { FileInputStream in = null; FileOutputStream out = null; try { in = new FileInputStream("xanadu.txt"); out = new FileOutputStream("outagain.txt"); int c; while ((c = in.read()) != -1) { out.write(c); } } finally { if (in != null) { in.close(); } if (out != null) { out.close(); } } } }
CopyBytes
spends most of its time in a simple loop that reads the input stream and writes the output stream, one byte at a time, as shown in the following figure.
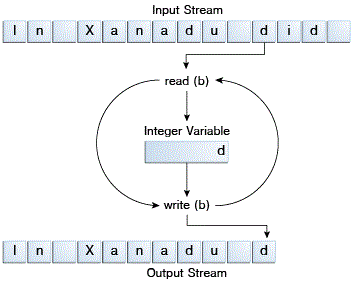
Simple byte stream input and output.
Notice that read()
returns an int
value. If the input is a stream of bytes, why doesn't read()
return a byte
value? Using a int
as a return type allows read()
to use -1 to indicate that it has reached the end of the stream.
Always Close Streams
Closing a stream when it's no longer needed is very important — so important that CopyBytes
uses a finally
block to guarantee that both streams will be closed even if an error occurs. This practice helps avoid serious resource leaks.
One possible error is that CopyBytes
was unable to open one or both files. When that happens, the stream variable corresponding to the file never changes from its initial null
value. That's why CopyBytes
makes sure that each stream variable contains an object reference before invoking close
.
When Not to Use Byte Streams
CopyBytes
seems like a normal program, but it actually represents a kind of low-level I/O that you should avoid. Since xanadu.txt
contains character data, the best approach is to use character streams, as discussed in the next section. There are also streams for more complicated data types. Byte streams should only be used for the most primitive I/O.
So why talk about byte streams? Because all other stream types are built on byte streams.
Character Streams
The Java platform stores character values using Unicode conventions. Character stream I/O automatically translates this internal format to and from the local character set. In Western locales, the local character set is usually an 8-bit superset of ASCII.
For most applications, I/O with character streams is no more complicated than I/O with byte streams. Input and output done with stream classes automatically translates to and from the local character set. A program that uses character streams in place of byte streams automatically adapts to the local character set and is ready for internationalization — all without extra effort by the programmer.
If internationalization isn't a priority, you can simply use the character stream classes without paying much attention to character set issues. Later, if internationalization becomes a priority, your program can be adapted without extensive recoding.
Using Character Streams
All character stream classes are descended from Reader
and Writer
. As with byte streams, there are character stream classes that specialize in file I/O: FileReader
and FileWriter
. The CopyCharacters
example illustrates these classes.
import java.io.FileReader; import java.io.FileWriter; import java.io.IOException; public class CopyCharacters { public static void main(String[] args) throws IOException { FileReader inputStream = null; FileWriter outputStream = null; try { inputStream = new FileReader("xanadu.txt"); outputStream = new FileWriter("characteroutput.txt"); int c; while ((c = inputStream.read()) != -1) { outputStream.write(c); } } finally { if (inputStream != null) { inputStream.close(); } if (outputStream != null) { outputStream.close(); } } } }
CopyCharacters
is very similar to CopyBytes
. The most important difference is that CopyCharacters
uses FileReader
and FileWriter
for input and output in place of FileInputStream
and FileOutputStream
. Notice that both CopyBytes
and CopyCharacters
use an int
variable to read to and write from. However, in CopyCharacters
, the int
variable holds a character value in its last 16 bits; in CopyBytes
, the int
variable holds a byte
value in its last 8 bits.
Character Streams that Use Byte Streams
Character streams are often "wrappers" for byte streams. The character stream uses the byte stream to perform the physical I/O, while the character stream handles translation between characters and bytes. FileReader
, for example, uses FileInputStream
, while FileWriter
uses FileOutputStream
.
There are two general-purpose byte-to-character "bridge" streams: InputStreamReader
and OutputStreamWriter
. Use them to create character streams when there are no prepackaged character stream classes that meet your needs. The sockets lesson in the networking trail shows how to create character streams from the byte streams provided by socket classes.
Line-Oriented I/O
Character I/O usually occurs in bigger units than single characters. One common unit is the line: a string of characters with a line terminator at the end. A line terminator can be a carriage-return/line-feed sequence ("\r\n"
), a single carriage-return ("\r"
), or a single line-feed ("\n"
). Supporting all possible line terminators allows programs to read text files created on any of the widely used operating systems.
Let's modify the CopyCharacters
example to use line-oriented I/O. To do this, we have to use two classes we haven't seen before, BufferedReader
and PrintWriter
. We'll explore these classes in greater depth in Buffered I/O and Formatting. Right now, we're just interested in their support for line-oriented I/O.
The CopyLines
example invokes BufferedReader.readLine
and PrintWriter.println
to do input and output one line at a time.
import java.io.FileReader; import java.io.FileWriter; import java.io.BufferedReader; import java.io.PrintWriter; import java.io.IOException; public class CopyLines { public static void main(String[] args) throws IOException { BufferedReader inputStream = null; PrintWriter outputStream = null; try { inputStream = new BufferedReader(new FileReader("xanadu.txt")); outputStream = new PrintWriter(new FileWriter("characteroutput.txt")); String l; while ((l = inputStream.readLine()) != null) { outputStream.println(l); } } finally { if (inputStream != null) { inputStream.close(); } if (outputStream != null) { outputStream.close(); } } } }
Invoking readLine
returns a line of text with the line. CopyLines
outputs each line using println
, which appends the line terminator for the current operating system. This might not be the same line terminator that was used in the input file.
There are many ways to structure text input and output beyond characters and lines. For more information, see Scanning and Formatting.