Learning Resources
Basics of Java
Variables
As you learned in the previous lesson, an object stores its state in fields.
int cadence = 0; int speed = 0; int gear = 1;
The What Is an Object? discussion introduced you to fields, but you probably have still a few questions, such as: What are the rules and conventions for naming a field? Besides int
, what other data types are there? Do fields have to be initialized when they are declared? Are fields assigned a default value if they are not explicitly initialized? We'll explore the answers to such questions in this lesson, but before we do, there are a few technical distinctions you must first become aware of. In the Java programming language, the terms "field" and "variable" are both used; this is a common source of confusion among new developers, since both often seem to refer to the same thing.
The Java programming language defines the following kinds of variables:
-
Instance Variables (Non-Static Fields) Technically speaking, objects store their individual states in "non-static fields", that is, fields declared without the
static
keyword. Non-static fields are also known as instance variables because their values are unique to each instance of a class (to each object, in other words); thecurrentSpeed
of one bicycle is independent from thecurrentSpeed
of another. -
Class Variables (Static Fields) A class variable is any field declared with the
static
modifier; this tells the compiler that there is exactly one copy of this variable in existence, regardless of how many times the class has been instantiated. A field defining the number of gears for a particular kind of bicycle could be marked asstatic
since conceptually the same number of gears will apply to all instances. The codestatic int numGears = 6;
would create such a static field. Additionally, the keywordfinal
could be added to indicate that the number of gears will never change. -
Local Variables Similar to how an object stores its state in fields, a method will often store its temporary state in local variables. The syntax for declaring a local variable is similar to declaring a field (for example,
int count = 0;
). There is no special keyword designating a variable as local; that determination comes entirely from the location in which the variable is declared — which is between the opening and closing braces of a method. As such, local variables are only visible to the methods in which they are declared; they are not accessible from the rest of the class. -
Parameters You've already seen examples of parameters, both in the
Bicycle
class and in themain
method of the "Hello World!" application. Recall that the signature for themain
method ispublic static void main(String[] args)
. Here, theargs
variable is the parameter to this method. The important thing to remember is that parameters are always classified as "variables" not "fields". This applies to other parameter-accepting constructs as well (such as constructors and exception handlers) that you'll learn about later in the tutorial.
Having said that, the remainder of this tutorial uses the following general guidelines when discussing fields and variables. If we are talking about "fields in general" (excluding local variables and parameters), we may simply say "fields". If the discussion applies to "all of the above", we may simply say "variables". If the context calls for a distinction, we will use specific terms (static field, local variables, etc.) as appropriate. You may also occasionally see the term "member" used as well. A type's fields, methods, and nested types are collectively called its members.
Naming
Every programming language has its own set of rules and conventions for the kinds of names that you're allowed to use, and the Java programming language is no different. The rules and conventions for naming your variables can be summarized as follows:
-
Variable names are case-sensitive. A variable's name can be any legal identifier — an unlimited-length sequence of Unicode letters and digits, beginning with a letter, the dollar sign "
$
", or the underscore character "_
". The convention, however, is to always begin your variable names with a letter, not "$
" or "_
". Additionally, the dollar sign character, by convention, is never used at all. You may find some situations where auto-generated names will contain the dollar sign, but your variable names should always avoid using it. A similar convention exists for the underscore character; while it's technically legal to begin your variable's name with "_
", this practice is discouraged. White space is not permitted. -
Subsequent characters may be letters, digits, dollar signs, or underscore characters. Conventions (and common sense) apply to this rule as well. When choosing a name for your variables, use full words instead of cryptic abbreviations. Doing so will make your code easier to read and understand. In many cases it will also make your code self-documenting; fields named
cadence
,speed
, andgear
, for example, are much more intuitive than abbreviated versions, such ass
,c
, andg
. Also keep in mind that the name you choose must not be a keyword or reserved word. -
If the name you choose consists of only one word, spell that word in all lowercase letters. If it consists of more than one word, capitalize the first letter of each subsequent word. The names
gearRatio
andcurrentGear
are prime examples of this convention. If your variable stores a constant value, such asstatic final int NUM_GEARS = 6
, the convention changes slightly, capitalizing every letter and separating subsequent words with the underscore character. By convention, the underscore character is never used elsewhere.
Primitive Data Types
The Java programming language is statically-typed, which means that all variables must first be declared before they can be used. This involves stating the variable's type and name, as you've already seen:
int gear = 1;
Doing so tells your program that a field named "gear" exists, holds numerical data, and has an initial value of "1". A variable's data type determines the values it may contain, plus the operations that may be performed on it. In addition to int
, the Java programming language supports seven other primitive data types. A primitive type is predefined by the language and is named by a reserved keyword. Primitive values do not share state with other primitive values. The eight primitive data types supported by the Java programming language are:
-
byte: The
byte
data type is an 8-bit signed two's complement integer. It has a minimum value of -128 and a maximum value of 127 (inclusive). Thebyte
data type can be useful for saving memory in large arrays, where the memory savings actually matters. They can also be used in place ofint
where their limits help to clarify your code; the fact that a variable's range is limited can serve as a form of documentation. -
short: The
short
data type is a 16-bit signed two's complement integer. It has a minimum value of -32,768 and a maximum value of 32,767 (inclusive). As withbyte
, the same guidelines apply: you can use ashort
to save memory in large arrays, in situations where the memory savings actually matters. -
int: The
int
data type is a 32-bit signed two's complement integer. It has a minimum value of -2,147,483,648 and a maximum value of 2,147,483,647 (inclusive). For integral values, this data type is generally the default choice unless there is a reason (like the above) to choose something else. This data type will most likely be large enough for the numbers your program will use, but if you need a wider range of values, uselong
instead. -
long: The
long
data type is a 64-bit signed two's complement integer. It has a minimum value of -9,223,372,036,854,775,808 and a maximum value of 9,223,372,036,854,775,807 (inclusive). Use this data type when you need a range of values wider than those provided byint
. -
float: The
float
data type is a single-precision 32-bit IEEE 754 floating point. Its range of values is beyond the scope of this discussion, but is specified in the Floating-Point Types, Formats, and Values section of the Java Language Specification. As with the recommendations forbyte
andshort
, use afloat
(instead ofdouble
) if you need to save memory in large arrays of floating point numbers. This data type should never be used for precise values, such as currency. For that, you will need to use the java.math.BigDecimal class instead. Numbers and Strings coversBigDecimal
and other useful classes provided by the Java platform. -
double: The
double
data type is a double-precision 64-bit IEEE 754 floating point. Its range of values is beyond the scope of this discussion, but is specified in the Floating-Point Types, Formats, and Values section of the Java Language Specification. For decimal values, this data type is generally the default choice. As mentioned above, this data type should never be used for precise values, such as currency. -
boolean: The
boolean
data type has only two possible values:true
andfalse
. Use this data type for simple flags that track true/false conditions. This data type represents one bit of information, but its "size" isn't something that's precisely defined. -
char: The
char
data type is a single 16-bit Unicode character. It has a minimum value of'\u0000'
(or 0) and a maximum value of'\uffff'
(or 65,535 inclusive).
In addition to the eight primitive data types listed above, the Java programming language also provides special support for character strings via the java.lang.String class. Enclosing your character string within double quotes will automatically create a new String
object; for example, String s = "this is a string";
. String
objects are immutable, which means that once created, their values cannot be changed. The String
class is not technically a primitive data type, but considering the special support given to it by the language, you'll probably tend to think of it as such. You'll learn more about the String
class in Simple Data Objects
Default Values
It's not always necessary to assign a value when a field is declared. Fields that are declared but not initialized will be set to a reasonable default by the compiler. Generally speaking, this default will be zero or null
, depending on the data type. Relying on such default values, however, is generally considered bad programming style.
The following chart summarizes the default values for the above data types.
Data Type | Default Value (for fields) |
---|---|
byte | 0 |
short | 0 |
int | 0 |
long | 0L |
float | 0.0f |
double | 0.0d |
char | '\u0000' |
String (or any object) | null |
boolean | false |
Local variables are slightly different; the compiler never assigns a default value to an uninitialized local variable. If you cannot initialize your local variable where it is declared, make sure to assign it a value before you attempt to use it. Accessing an uninitialized local variable will result in a compile-time error.
Literals
You may have noticed that the new
keyword isn't used when initializing a variable of a primitive type. Primitive types are special data types built into the language; they are not objects created from a class. A literal is the source code representation of a fixed value; literals are represented directly in your code without requiring computation. As shown below, it's possible to assign a literal to a variable of a primitive type:
boolean result = true; char capitalC = 'C'; byte b = 100; short s = 10000; int i = 100000;
Integer Literals
An integer literal is of type long
if it ends with the letter L
or l
; otherwise it is of type int
. It is recommended that you use the upper case letter L
because the lower case letter l
is hard to distinguish from the digit 1
.
Values of the integral types byte
, short
, int
, and long
can be created from int
literals. Values of type long
that exceed the range of int
can be created from long
literals. Integer literals can be expressed by these number systems:
- Decimal: Base 10, whose digits consists of the numbers 0 through 9; this is the number system you use every day
- Hexadecimal: Base 16, whose digits consist of the numbers 0 through 9 and the letters A through F
- Binary: Base 2, whose digits consists of the numbers 0 and 1 (you can create binary literals in Java SE 7 and later)
For general-purpose programming, the decimal system is likely to be the only number system you'll ever use. However, if you need to use another number system, the following example shows the correct syntax. The prefix 0x
indicates hexadecimal and 0b
indicates binary:
// The number 26, in decimal int decVal = 26; // The number 26, in hexadecimal int hexVal = 0x1a; // The number 26, in binary int binVal = 0b11010;
Floating-Point Literals
A floating-point literal is of type float
if it ends with the letter F
or f
; otherwise its type is double
and it can optionally end with the letter D
or d
.
The floating point types (float
and double
) can also be expressed using E or e (for scientific notation), F or f (32-bit float literal) and D or d (64-bit double literal; this is the default and by convention is omitted).
double d1 = 123.4; // same value as d1, but in scientific notation double d2 = 1.234e2; float f1 = 123.4f;
Character and String Literals
Literals of types char
and String
may contain any Unicode (UTF-16) characters. If your editor and file system allow it, you can use such characters directly in your code. If not, you can use a "Unicode escape" such as '\u0108'
(capital C with circumflex), or "S\u00ED Se\u00F1or"
(Sí Señor in Spanish). Always use 'single quotes' for char
literals and "double quotes" for String
literals. Unicode escape sequences may be used elsewhere in a program (such as in field names, for example), not just in char
or String
literals.
The Java programming language also supports a few special escape sequences for char
and String
literals: \b
(backspace), \t
(tab), \n
(line feed), \f
(form feed), \r
(carriage return), \"
(double quote), \'
(single quote), and \\
(backslash).
There's also a special null
literal that can be used as a value for any reference type. null
may be assigned to any variable, except variables of primitive types. There's little you can do with a null
value beyond testing for its presence. Therefore, null
is often used in programs as a marker to indicate that some object is unavailable.
Finally, there's also a special kind of literal called a class literal, formed by taking a type name and appending ".class"
; for example, String.class
. This refers to the object (of type Class
) that represents the type itself.
Using Underscore Characters in Numeric Literals
In Java SE 7 and later, any number of underscore characters (_
) can appear anywhere between digits in a numerical literal. This feature enables you, for example. to separate groups of digits in numeric literals, which can improve the readability of your code.
For instance, if your code contains numbers with many digits, you can use an underscore character to separate digits in groups of three, similar to how you would use a punctuation mark like a comma, or a space, as a separator.
The following example shows other ways you can use the underscore in numeric literals:
long creditCardNumber = 1234_5678_9012_3456L; long socialSecurityNumber = 999_99_9999L; float pi = 3.14_15F; long hexBytes = 0xFF_EC_DE_5E; long hexWords = 0xCAFE_BABE; long maxLong = 0x7fff_ffff_ffff_ffffL; byte nybbles = 0b0010_0101; long bytes = 0b11010010_01101001_10010100_10010010;
You can place underscores only between digits; you cannot place underscores in the following places:
- At the beginning or end of a number
- Adjacent to a decimal point in a floating point literal
-
Prior to an
F
orL
suffix - In positions where a string of digits is expected
The following examples demonstrate valid and invalid underscore placements (which are highlighted) in numeric literals:
// Invalid: cannot put underscores // adjacent to a decimal point float pi1 = 3_.1415F; // Invalid: cannot put underscores // adjacent to a decimal point float pi2 = 3._1415F; // Invalid: cannot put underscores // prior to an L suffix long socialSecurityNumber1 = 999_99_9999_L; // This is an identifier, not // a numeric literal int x1 = _52; // OK (decimal literal) int x2 = 5_2; // Invalid: cannot put underscores // At the end of a literal int x3 = 52_; // OK (decimal literal) int x4 = 5_______2; // Invalid: cannot put underscores // in the 0x radix prefix int x5 = 0_x52; // Invalid: cannot put underscores // at the beginning of a number int x6 = 0x_52; // OK (hexadecimal literal) int x7 = 0x5_2; // Invalid: cannot put underscores // at the end of a number int x8 = 0x52_;
Arrays
An array is a container object that holds a fixed number of values of a single type. The length of an array is established when the array is created. After creation, its length is fixed. You've seen an example of arrays already, in the main
method of the "Hello World!" application. This section discusses arrays in greater detail.
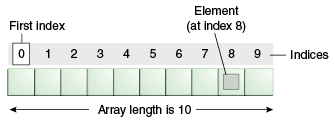
An array of ten elements
Each item in an array is called an element, and each element is accessed by its numerical index. As shown in the above illustration, numbering begins with 0. The 9th element, for example, would therefore be accessed at index 8.
The following program, ArrayDemo
, creates an array of integers, puts some values in it, and prints each value to standard output.
class ArrayDemo { public static void main(String[] args) { // declares an array of integers int[] anArray; // allocates memory for 10 integers anArray = new int[10]; // initialize first element anArray[0] = 100; // initialize second element anArray[1] = 200; // etc. anArray[2] = 300; anArray[3] = 400; anArray[4] = 500; anArray[5] = 600; anArray[6] = 700; anArray[7] = 800; anArray[8] = 900; anArray[9] = 1000; System.out.println("Element at index 0: " + anArray[0]); System.out.println("Element at index 1: " + anArray[1]); System.out.println("Element at index 2: " + anArray[2]); System.out.println("Element at index 3: " + anArray[3]); System.out.println("Element at index 4: " + anArray[4]); System.out.println("Element at index 5: " + anArray[5]); System.out.println("Element at index 6: " + anArray[6]); System.out.println("Element at index 7: " + anArray[7]); System.out.println("Element at index 8: " + anArray[8]); System.out.println("Element at index 9: " + anArray[9]); } }
The output from this program is:
Element at index 0: 100 Element at index 1: 200 Element at index 2: 300 Element at index 3: 400 Element at index 4: 500 Element at index 5: 600 Element at index 6: 700 Element at index 7: 800 Element at index 8: 900 Element at index 9: 1000
In a real-world programming situation, you'd probably use one of the supported looping constructs to iterate through each element of the array, rather than write each line individually as shown above. However, this example clearly illustrates the array syntax. You'll learn about the various looping constructs (for
, while
, and do-while
) in the Control Flow section.
Declaring a Variable to Refer to an Array
The above program declares anArray
with the following line of code:
// declares an array of integers int[] anArray;
Like declarations for variables of other types, an array declaration has two components: the array's type and the array's name. An array's type is written as type[]
, where type
is the data type of the contained elements; the square brackets are special symbols indicating that this variable holds an array. The size of the array is not part of its type (which is why the brackets are empty). An array's name can be anything you want, provided that it follows the rules and conventions as previously discussed in the naming section. As with variables of other types, the declaration does not actually create an array — it simply tells the compiler that this variable will hold an array of the specified type.
Similarly, you can declare arrays of other types:
byte[] anArrayOfBytes; short[] anArrayOfShorts; long[] anArrayOfLongs; float[] anArrayOfFloats; double[] anArrayOfDoubles; boolean[] anArrayOfBooleans; char[] anArrayOfChars; String[] anArrayOfStrings;
You can also place the square brackets after the array's name:
// this form is discouraged float anArrayOfFloats[];
However, convention discourages this form; the brackets identify the array type and should appear with the type designation.
Creating, Initializing, and Accessing an Array
One way to create an array is with the new
operator. The next statement in the ArrayDemo
program allocates an array with enough memory for ten integer elements and assigns the array to the anArray
variable.
// create an array of integers anArray = new int[10];
If this statement were missing, the compiler would print an error like the following, and compilation would fail:
ArrayDemo.java:4: Variable anArray may not have been initialized.
The next few lines assign values to each element of the array:
anArray[0] = 100; // initialize first element anArray[1] = 200; // initialize second element anArray[2] = 300; // etc.
Each array element is accessed by its numerical index:
System.out.println("Element 1 at index 0: " + anArray[0]); System.out.println("Element 2 at index 1: " + anArray[1]); System.out.println("Element 3 at index 2: " + anArray[2]);
Alternatively, you can use the shortcut syntax to create and initialize an array:
int[] anArray = { 100, 200, 300, 400, 500, 600, 700, 800, 900, 1000 };
Here the length of the array is determined by the number of values provided between { and }.
You can also declare an array of arrays (also known as a multidimensional array) by using two or more sets of square brackets, such as String[][] names
. Each element, therefore, must be accessed by a corresponding number of index values.
In the Java programming language, a multidimensional array is simply an array whose components are themselves arrays. This is unlike arrays in C or Fortran. A consequence of this is that the rows are allowed to vary in length, as shown in the following MultiDimArrayDemo
program:
class MultiDimArrayDemo { public static void main(String[] args) { String[][] names = { {"Mr. ", "Mrs. ", "Ms. "}, {"Smith", "Jones"} }; // Mr. Smith System.out.println(names[0][0] + names[1][0]); // Ms. Jones System.out.println(names[0][2] + names[1][1]); } }
The output from this program is:
Mr. Smith Ms. Jones
Finally, you can use the built-in length
property to determine the size of any array. The code
System.out.println(anArray.length);
will print the array's size to standard output.
Copying Arrays
The System
class has an arraycopy
method that you can use to efficiently copy data from one array into another:
public static void arraycopy(Object src, int srcPos, Object dest, int destPos, int length)
The two Object
arguments specify the array to copy from and the array to copy to. The three int
arguments specify the starting position in the source array, the starting position in the destination array, and the number of array elements to copy.
The following program, ArrayCopyDemo
, declares an array of char
elements, spelling the word "decaffeinated". It uses arraycopy
to copy a subsequence of array components into a second array:
class ArrayCopyDemo { public static void main(String[] args) { char[] copyFrom = { 'd', 'e', 'c', 'a', 'f', 'f', 'e', 'i', 'n', 'a', 't', 'e', 'd' }; char[] copyTo = new char[7]; System.arraycopy(copyFrom, 2, copyTo, 0, 7); System.out.println(new String(copyTo)); } }
The output from this program is:
caffein
Operators
Now that you've learned how to declare and initialize variables, you probably want to know how to do something with them. Learning the operators of the Java programming language is a good place to start. Operators are special symbols that perform specific operations on one, two, or three operands, and then return a result.
As we explore the operators of the Java programming language, it may be helpful for you to know ahead of time which operators have the highest precedence. The operators in the following table are listed according to precedence order. The closer to the top of the table an operator appears, the higher its precedence. Operators with higher precedence are evaluated before operators with relatively lower precedence. Operators on the same line have equal precedence. When operators of equal precedence appear in the same expression, a rule must govern which is evaluated first. All binary operators except for the assignment operators are evaluated from left to right; assignment operators are evaluated right to left.
Operators | Precedence |
---|---|
postfix |
expr++ expr-- |
unary |
++expr --expr +expr -expr ~ ! |
multiplicative |
* / % |
additive |
+ - |
shift |
<< >> >>> |
relational |
< > <= >= instanceof |
equality |
== != |
bitwise AND |
& |
bitwise exclusive OR |
^ |
bitwise inclusive OR |
| |
logical AND |
&& |
logical OR |
|| |
ternary |
? : |
assignment |
= += -= *= /= %= &= ^= |= <<= >>= >>>= |
In general-purpose programming, certain operators tend to appear more frequently than others; for example, the assignment operator "=
" is far more common than the unsigned right shift operator ">>>
". With that in mind, the following discussion focuses first on the operators that you're most likely to use on a regular basis, and ends focusing on those that are less common. Each discussion is accompanied by sample code that you can compile and run. Studying its output will help reinforce what you've just learned.
Assignment, Arithmetic, and Unary Operators
The Simple Assignment Operator
One of the most common operators that you'll encounter is the simple assignment operator "=
". You saw this operator in the Bicycle class; it assigns the value on its right to the operand on its left:
int cadence = 0; int speed = 0; int gear = 1;
This operator can also be used on objects to assign object references, as discussed in Creating Objects.
The Arithmetic Operators
The Java programming language provides operators that perform addition, subtraction, multiplication, and division. There's a good chance you'll recognize them by their counterparts in basic mathematics. The only symbol that might look new to you is "%
", which divides one operand by another and returns the remainder as its result.
+ additive operator (also used for String concatenation) - subtraction operator * multiplication operator / division operator % remainder operator
The following program,ArithmeticDemo
, tests the arithmetic operators.
class ArithmeticDemo { public static void main (String[] args){ // result is now 3 int result = 1 + 2; System.out.println(result); // result is now 2 result = result - 1; System.out.println(result); // result is now 4 result = result * 2; System.out.println(result); // result is now 2 result = result / 2; System.out.println(result); // result is now 10 result = result + 8; // result is now 3 result = result % 7; System.out.println(result); } }
You can also combine the arithmetic operators with the simple assignment operator to create compound assignments. For example, x+=1;
and x=x+1;
both increment the value of x
by 1.
The +
operator can also be used for concatenating (joining) two strings together, as shown in the following ConcatDemo
program:
class ConcatDemo { public static void main(String[] args){ String firstString = "This is"; String secondString = " a concatenated string."; String thirdString = firstString+secondString; System.out.println(thirdString); } }
By the end of this program, the variable thirdString
contains "This is a concatenated string.", which gets printed to standard output.
The Unary Operators
The unary operators require only one operand; they perform various operations such as incrementing/decrementing a value by one, negating an expression, or inverting the value of a boolean.
+ Unary plus operator; indicates positive value (numbers are positive without this, however) - Unary minus operator; negates an expression ++ Increment operator; increments a value by 1 -- Decrement operator; decrements a value by 1 ! Logical complement operator; inverts the value of a boolean
The following program, UnaryDemo
, tests the unary operators:
class UnaryDemo { public static void main(String[] args){ // result is now 1 int result = +1; System.out.println(result); // result is now 0 result--; System.out.println(result); // result is now 1 result++; System.out.println(result); // result is now -1 result = -result; System.out.println(result); boolean success = false; // false System.out.println(success); // true System.out.println(!success); } }
The increment/decrement operators can be applied before (prefix) or after (postfix) the operand. The code result++;
and ++result;
will both end in result
being incremented by one. The only difference is that the prefix version (++result
) evaluates to the incremented value, whereas the postfix version (result++
) evaluates to the original value. If you are just performing a simple increment/decrement, it doesn't really matter which version you choose. But if you use this operator in part of a larger expression, the one that you choose may make a significant difference.
The following program, PrePostDemo
, illustrates the prefix/postfix unary increment operator:
class PrePostDemo { public static void main(String[] args){ int i = 3; i++; // prints 4 System.out.println(i); ++i; // prints 5 System.out.println(i); // prints 6 System.out.println(++i); // prints 6 System.out.println(i++); // prints 7 System.out.println(i); } }
Equality, Relational, and Conditional Operators
The Equality and Relational Operators
The equality and relational operators determine if one operand is greater than, less than, equal to, or not equal to another operand. The majority of these operators will probably look familiar to you as well. Keep in mind that you must use "==
", not "=
", when testing if two primitive values are equal.
== equal to != not equal to > greater than >= greater than or equal to < less than <= less than or equal to
The following program,ComparisonDemo
, tests the comparison operators:
class ComparisonDemo { public static void main(String[] args){ int value1 = 1; int value2 = 2; if(value1 == value2) System.out.println("value1 == value2"); if(value1 != value2) System.out.println("value1 != value2"); if(value1 > value2) System.out.println("value1 > value2"); if(value1 < value2) System.out.println("value1 < value2"); if(value1 <= value2) System.out.println("value1 <= value2"); } }
Output:
value1 != value2 value1 < value2 value1 <= value2
The Conditional Operators
The &&
and ||
operators perform Conditional-AND and Conditional-OR operations on two boolean expressions. These operators exhibit "short-circuiting" behavior, which means that the second operand is evaluated only if needed.
&& Conditional-AND || Conditional-OR
The following program,ConditionalDemo1
, tests these operators:
class ConditionalDemo1 { public static void main(String[] args){ int value1 = 1; int value2 = 2; if((value1 == 1) && (value2 == 2)) System.out.println("value1 is 1 AND value2 is 2"); if((value1 == 1) || (value2 == 1)) System.out.println("value1 is 1 OR value2 is 1"); } }
Another conditional operator is ?:
, which can be thought of as shorthand for an if-then-else
statement (discussed in the Control Flow Statements section of this lesson). This operator is also known as the ternary operator because it uses three operands. In the following example, this operator should be read as: "If someCondition
is true
, assign the value of value1
to result
. Otherwise, assign the value of value2
to result
."
The following program, ConditionalDemo2
, tests the ?:
operator:
class ConditionalDemo2 { public static void main(String[] args){ int value1 = 1; int value2 = 2; int result; boolean someCondition = true; result = someCondition ? value1 : value2; System.out.println(result); } }
Because someCondition
is true, this program prints "1" to the screen. Use the ?:
operator instead of an if-then-else
statement if it makes your code more readable; for example, when the expressions are compact and without side-effects (such as assignments).
The Type Comparison Operator instanceof
The instanceof
operator compares an object to a specified type. You can use it to test if an object is an instance of a class, an instance of a subclass, or an instance of a class that implements a particular interface.
The following program, InstanceofDemo
, defines a parent class (named Parent
), a simple interface (named MyInterface
), and a child class (named Child
) that inherits from the parent and implements the interface.
class InstanceofDemo { public static void main(String[] args) { Parent obj1 = new Parent(); Parent obj2 = new Child(); System.out.println("obj1 instanceof Parent: " + (obj1 instanceof Parent)); System.out.println("obj1 instanceof Child: " + (obj1 instanceof Child)); System.out.println("obj1 instanceof MyInterface: " + (obj1 instanceof MyInterface)); System.out.println("obj2 instanceof Parent: " + (obj2 instanceof Parent)); System.out.println("obj2 instanceof Child: " + (obj2 instanceof Child)); System.out.println("obj2 instanceof MyInterface: " + (obj2 instanceof MyInterface)); } } class Parent {} class Child extends Parent implements MyInterface {} interface MyInterface {}
Output:
obj1 instanceof Parent: true obj1 instanceof Child: false obj1 instanceof MyInterface: false obj2 instanceof Parent: true obj2 instanceof Child: true obj2 instanceof MyInterface: true
When using the instanceof
operator, keep in mind that null
is not an instance of anything.
Bitwise and Bit Shift Operators
The Java programming language also provides operators that perform bitwise and bit shift operations on integral types. The operators discussed in this section are less commonly used. Therefore, their coverage is brief; the intent is to simply make you aware that these operators exist.
The unary bitwise complement operator "~
" inverts a bit pattern; it can be applied to any of the integral types, making every "0" a "1" and every "1" a "0". For example, a byte
contains 8 bits; applying this operator to a value whose bit pattern is "00000000" would change its pattern to "11111111".
The signed left shift operator "<<
" shifts a bit pattern to the left, and the signed right shift operator ">>
" shifts a bit pattern to the right. The bit pattern is given by the left-hand operand, and the number of positions to shift by the right-hand operand. The unsigned right shift operator ">>>
" shifts a zero into the leftmost position, while the leftmost position after ">>"
depends on sign extension.
The bitwise &
operator performs a bitwise AND operation.
The bitwise ^
operator performs a bitwise exclusive OR operation.
The bitwise |
operator performs a bitwise inclusive OR operation.
The following program, BitDemo
, uses the bitwise AND operator to print the number "2" to standard output.
class BitDemo { public static void main(String[] args) { int bitmask = 0x000F; int val = 0x2222; // prints "2" System.out.println(val & bitmask); } }
Expressions, Statements, and Blocks
Now that you understand variables and operators, it's time to learn about expressions, statements, and blocks. Operators may be used in building expressions, which compute values; expressions are the core components of statements; statements may be grouped into blocks.
Expressions
An expression is a construct made up of variables, operators, and method invocations, which are constructed according to the syntax of the language, that evaluates to a single value. You've already seen examples of expressions, illustrated in bold below:
int cadence = 0;
anArray[0] = 100;
System.out.println("Element 1 at index 0: " + anArray[0]);
int result = 1 + 2; // result is now 3
if (value1 == value2) System.out.println("value1 == value2");
The data type of the value returned by an expression depends on the elements used in the expression. The expression cadence = 0
returns an int
because the assignment operator returns a value of the same data type as its left-hand operand; in this case, cadence
is an int
. As you can see from the other expressions, an expression can return other types of values as well, such as boolean
or String
.
The Java programming language allows you to construct compound expressions from various smaller expressions as long as the data type required by one part of the expression matches the data type of the other. Here's an example of a compound expression:
1 * 2 * 3
In this particular example, the order in which the expression is evaluated is unimportant because the result of multiplication is independent of order; the outcome is always the same, no matter in which order you apply the multiplications. However, this is not true of all expressions. For example, the following expression gives different results, depending on whether you perform the addition or the division operation first:
x + y / 100 // ambiguous
You can specify exactly how an expression will be evaluated using balanced parenthesis: ( and ). For example, to make the previous expression unambiguous, you could write the following:
(x + y) / 100 // unambiguous, recommended
If you don't explicitly indicate the order for the operations to be performed, the order is determined by the precedence assigned to the operators in use within the expression. Operators that have a higher precedence get evaluated first. For example, the division operator has a higher precedence than does the addition operator. Therefore, the following two statements are equivalent:
x + y / 100 x + (y / 100) // unambiguous, recommended
When writing compound expressions, be explicit and indicate with parentheses which operators should be evaluated first. This practice makes code easier to read and to maintain.
Statements
Statements are roughly equivalent to sentences in natural languages. A statement forms a complete unit of execution. The following types of expressions can be made into a statement by terminating the expression with a semicolon (;
).
- Assignment expressions
-
Any use of
++
or--
- Method invocations
- Object creation expressions
Such statements are called expression statements. Here are some examples of expression statements.
// assignment statement aValue = 8933.234; // increment statement aValue++; // method invocation statement System.out.println("Hello World!"); // object creation statement Bicycle myBike = new Bicycle();
In addition to expression statements, there are two other kinds of statements: declaration statements and control flow statements. A declaration statement declares a variable. You've seen many examples of declaration statements already:
// declaration statement double aValue = 8933.234;
Finally, control flow statements regulate the order in which statements get executed. You'll learn about control flow statements in the next section, Control Flow Statements
Blocks
A block is a group of zero or more statements between balanced braces and can be used anywhere a single statement is allowed. The following example, BlockDemo
, illustrates the use of blocks:
class BlockDemo { public static void main(String[] args) { boolean condition = true; if (condition) { // begin block 1 System.out.println("Condition is true."); } // end block one else { // begin block 2 System.out.println("Condition is false."); } // end block 2 } }