App Events help you understand the makeup of people who engage with your app and measure and reach specific sets of your users with Facebook mobile app ads. This is done by logging events from your app via Facebook SDK for Swift. The event can be one of 14 predefined events such as added to cart in a commerce app, achieved level in a game, or any custom events you define.
Prerequisites
Before including the code to measure events, you’ll need to register your app with Facebook and install the Facebook SDK.
Implementation
Facebook’s SDK provides helper methods for app activation and the built-in types of events.
Logging app activations – Logging app activations as an app event enables most other functionality and should be the first thing that you add to your app. The SDK provides a helper method to log app activation. By logging an activation event, you can observe how frequently users activate your app, how much time they spend using it, and view other demographic information through Facebook Analytics.
Insert this code into your app delegate’s applicationDidBecomeActive method:
import FacebookCore
func applicationDidBecomeActive(application: UIApplication) {
// Call the ‘activate’ method to log an app event for use
// in analytics and advertising reporting.
AppEventsLogger.activate(application)
// …
}
The AppEventsLogger.activate method is the preferred way to log app activations even though there’s an event that you can send manually via the SDK. The helper method performs a few other tasks that are necessary for proper accounting for Mobile App Install Ads.
Other event types – Much like logging app activations, the Facebook SDK provides a helper methods to log other common events with a single line of code.
For example, a purchase of USD $4.32, can be logged with:
AppEventsLogger.log(.Purchased(amount: 4.32, currency: “USD”))
For purchases, the currency specification is expected to be an ISO 4217 currency code. This is used to determine a uniform value for use in ads optimization.
Automatic In-App Purchase Logging – Whenever possible, we suggest that you log purchases manually. However, Facebook has made it possible to automatically log app events related to in-app purchases. To enable automatic purchase logging, enable the Automatic In-App Purchase switch in the iOS settings section of the app’s dashboard and call the FBSDKAppEvents.activateApp method during app activation. Automatic logging requires the Facebook SDK for iOS version 3.22 or higher.
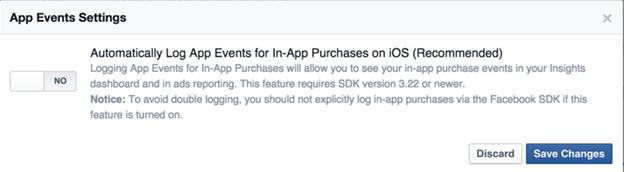
The maximum number of different event names is 1000. No new event types will be logged once this cap is hit and if you exceed this limit you may see a 100 Invalid parameter error when logging. However, it is possible to deactivate obsolete events.
Logging Events Manually
For all other types of events, use the log method with one of the event types defined in the AppEvent struct:
AppEventsLogger.log(
.AddedToCart(
contentType: “product”,
contentId: “HDFU-8452”,
currency: “USD”))
For any of the built-in app events type, you can also specify a set of additional parameters and a valueToSum property which is an arbitrary number that can represent any value like a price or a quantity. When reported, all of the valueToSum properties will be summed together in Facebook Analytics. For example, if 10 people each purchased one item that cost $10 and it was passed in valueToSum, then the Analytics report would show a value of $100.
Custom App Events
To log a custom event, just pass the name of the event as a string along with any additional parameters:
AppEventsLogger.log(“YouWereEatenByAGrue”)
Verifying Event Logging in Facebook Analytics
If you are an admin or developer of an app, please see our documentation on debugging events in Facebook Analytics to learn how to verify that events are being logged.
Enabling Debug Logs – Enable debug logs to verify client-side app event usage using the following code. The debug logs contain detailed requests and responses formatted in JSON.
SDKSettings.enableLoggingBehavior(.AppEvents)
Debug logging is intended for testing only and should not be enabled in production apps.
Facebook Analytics
Enabling app events means that you can automatically start using Facebook Analytics. This analytics channel provides demographic info about the people using your app, offers tools for better understanding the flows people follow in your app, and lets you compare cohorts of different kinds of people performing the same actions.
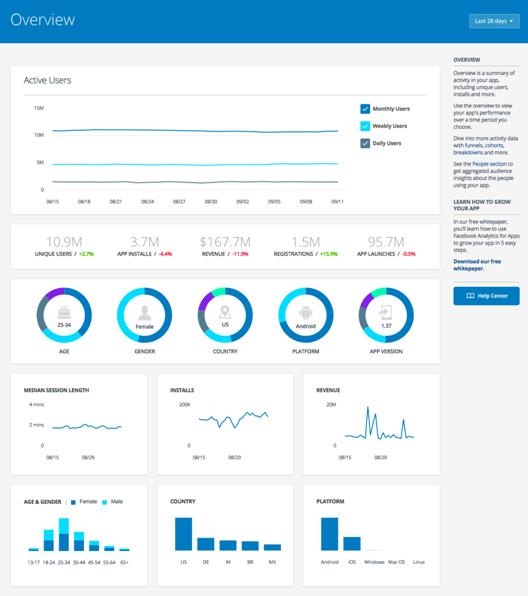
Facebook Ads
Mobile App Ad Performance – You can use app events to better understand the engagement and return on investment (ROI) coming from your mobile ads on Facebook. If you’ve set up app events, they will be reported as different types of actions in Ads Manager so you can see which ads and campaigns resulted in what actions within your app. For example, you may see that a particular ad resulted in 10 total actions. Those actions could be 8 checkouts and 2 registrations, which will be specified in Ads Manager.
Mobile App Ad Targeting – Measuring app events also opens up an easier way to reach your existing app users for mobile app ads. After integrating app events, you will be able to reach your existing users, specific subsets of users based on what actions they have taken within your app, or even percentages of your most active users. For instance you can run ads to bring recent purchasers back into your app in order to complete more purchases or to your top 25% of purchasers. You can choose an audience to reach based on the app events you are measuring in your app.
Ads Attribution – Once you’ve implemented app events into your iOS app, the app events you’ve added will automatically be tracked when you run Facebook mobile app ads for installs or mobile app ads for engagement and conversion.
If using Power Editor to set up your ad, please ensure you use the default tracking settings. If you manually configure tracking settings where tracking_spec is present, use the following code, replacing {app_id} with your app’s Facebook app ID.
{“action.type” : [“app_custom_event”],
“application” : [{app_id}]}
If you have set up app events, they will be reported as different types of actions in Ads Manager. For example, you may see that a particular ad resulted in 10 total actions. Those actions could be 8 checkouts and 2 registrations, which will be specified in Ads Manager.
Data Control
The Facebook SDK offers a tool to give your users control over how app events data is used by the Facebook ads system. Our Platform Policy requires that you provide users with an option to opt out of sharing this info with Facebook. We recommend that you use our SDK tools to offer the opt-out option. Facebook also respects device-level controls where available, including the Advertising Identifier control on iOS 6 and beyond.
limitedEventAndDataUsage behavior – If the user has set the limitedEventAndDataUsage flag to true, your app will continue to send this data to Facebook, but Facebook will not use the data to serve targeted ads. Facebook may continue to use the information for other purposes, including frequency capping, conversion events, estimating the number of unique users, security and fraud detection, and debugging.
SDKSettings.limitedEventAndDataUsage = true
The limitedEventAndDataUsage setting will persist across app sessions. To use this property, you can match your opt-out user interface to the value of the limitedEventAndDataUsage flag.
Data Sharing – App event data you share with Facebook will not be shared with advertisers or other third parties unless we have your permission or are required to do so by law.