The Facebook SDK for iOS is the easiest way to integrate your iOS app with Facebook. It enables:
- Facebook Login – Authenticate people with their Facebook credentials.
- Share and Send dialogs – Enable sharing content from your app to Facebook.
- App Events – Log events in your application.
- Graph API – Read and write to Graph API.
You have two ways to set up your app to use the Facebook SDK:
- By using Quick Start.
- By setting up manually
Using QuickStart
To get a Facebook App ID, configure your app’s settings, and import the Facebook SDK, access on the link – https://developers.facebook.com/quickstarts
Manual Configuration and Installation
Steps to configure iOS SDK for Facebook manually, are
Step 1: Configure Facebook App Settings for iOS
- Open the Facebook App Dashboard by clicking on the button below and selecting your application.
- Open Facebook App Dashboard
- Select Settings from the left navigation.
- Click Add Platform at the bottom of the page and select iOS.
- Locate your bundle identifier in Xcode and copy it to your clipboard.
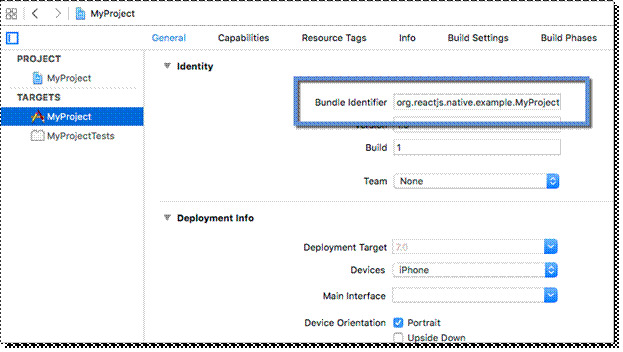
- Return to the App Dashboard and paste your bundle identifier into the Bundle ID field.
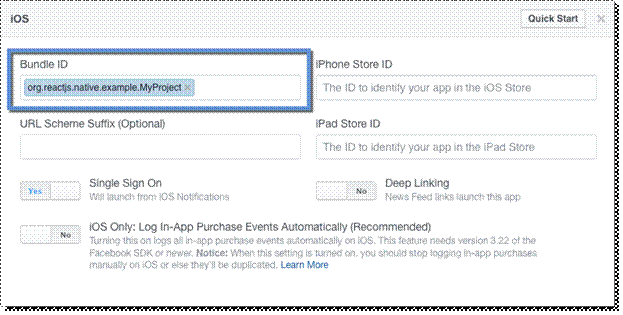
- Enable Single Sign On.
- Click Save Changes at the bottom of the App Dashboard window.
Step 2: Download Facebook SDK for iOS
- Download the SDK from the link – https://developers.facebook.com/docs/ios/downloads
- Download iOS SDK
- Unzip the archive to ~/Documents/FacebookSDK.
Step 3: Add SDK to Project
To add the SDK in Xcode:
- Open your application’s Xcode project.
- If you don’t have a Frameworks group in your project, create one.
- Open ~/Documents/FacebookSDK using Finder.
- Drag the Bolts.framework, FBSDKCoreKit.framework, FBSDKLoginKit.framework, and FBSDKShareKit.framework files into the Frameworks group of Xcode’s Project Navigator. When prompted, select “Copy items if needed” and continue.
Step 4: Configure Info.plist
- In Xcode, right-click your project’s Info.plist file and select Open As -> Source Code.
- Insert the following XML snippet into the body of your file just before the final </dict> element.
<key>CFBundleURLTypes</key>
<array>
<dict>
<key>CFBundleURLSchemes</key>
<array>
<string>fb{your-app-id}</string>
</array>
</dict>
</array>
<key>FacebookAppID</key>
<string>{your-app-id}</string>
<key>FacebookDisplayName</key>
<string>{your-app-name}</string>
<key>LSApplicationQueriesSchemes</key>
<array>
<string>fbapi</string>
<string>fb-messenger-share-api</string>
<string>fbauth2</string>
<string>fbshareextension</string>
</array>
- Replace {your-app-id}, and {your-app-name} with your app’s App’s ID and name found on the Facebook App Dashboard.
Step 5: Connect App Delegate
To post-process the results from actions that require you to switch to the native Facebook app or Safari, such as Facebook Login or Facebook Dialogs, you need to connect your AppDelegate class to the FBSDKApplicationDelegate object. To accomplish this, add the following code to your AppDelegate.m file.
// AppDelegate.m
#import <FBSDKCoreKit/FBSDKCoreKit.h>
– (BOOL)application:(UIApplication *)application
didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
[[FBSDKApplicationDelegate sharedInstance] application:applicationdidFinishLaunchingWithOptions:launchOptions];
// Add any custom logic here.
return YES;
}
– (BOOL)application:(UIApplication *)application
openURL:(NSURL *)url
options:(NSDictionary<UIApplicationOpenURLOptionsKey,id> *)options {
BOOL handled = [[FBSDKApplicationDelegate sharedInstance] application:application
openURL:url
sourceApplication:options[UIApplicationOpenURLOptionsSourceApplicationKey]
annotation:options[UIApplicationOpenURLOptionsAnnotationKey]
];
// Add any custom logic here.
return handled;
}
In the sample implementation of -application:openURL:sourceApplication:annotation: above, the call to FBSDKApplicationDelegate is required for deferred deep linking to work correctly.
Step 6: Add App Events
Now that the SDK is installed and configured, the easiest way to test it is to add App Events to your app. App Events help you understand how people are using your app. This is done by logging events via one of 14 predefined events such as added to cart in a commerce app or level achieved in a game. You can even define your own custom events.
Log App Activations – To see how many people are using your application, log app activations by adding the following code to your AppDelegate.m file.
// AppDelegate.m
#import <FBSDKCoreKit/FBSDKCoreKit.h>
– (void)applicationDidBecomeActive:(UIApplication *)application {
[FBSDKAppEvents activateApp];}
To verify logging:
- Compile and run your app.
- Go to the Facebook Analytics Dashboard and select your app.
- Open Facebook Analytics
- From the menu on the left, select Activity -> Events.
There will be a short delay before your activations show on the event dashboard. If you don’t see anything, wait a minute and refresh the page.
When you use the Facebook SDK, events in your app are automatically logged and collected for Facebook Analytics unless you disable automatic event logging.
Create a Simulator Build (for App Review)
For iOS apps, you should generate a simulator binary package and upload it for us to use as part of the review process. To generate a simulator build:
Step 1 – Run App in Simulator
Run your app in your Xcode iPhone 5 simulator. This automatically creates a simulator build in Xcode’s DerivedData cache.
Step 2 – ZIP Simulator Build
Zip the simulator build via the following command:
ditto -ck –sequesterRsrc –keepParent `ls -1 -d -t ~/Library/Developer/Xcode/DerivedData/*/Build/Products/*-iphonesimulator/*.app | head -n 1` path/to/YourApp.zip
Step 3 – Step: Verify Build
You can verify the simulator build by using the ios-sim utility Command-line App Launcher for Simulator. Once installed run:
ios-sim launch /path/to/your-app.app
Step 4 – Submit for Review
Submit file zip file (e.g. YourApp.zip) using your app dashboard. Please note that we delete the simulator build you provide three weeks after your submission has been actioned, i.e. reviewed or canceled.
Advanced App Configuration
Optionally, if you want all the SDK classes to work out of the box you can also add the -ObjC linker flag to your project.
If you to do this you increase the size of your app’s executable since the due to additional SDK object code loads into your application.
You can use one Facebook app ID in multiple iOS apps, for example, between free and paid versions of your app. Define a URL scheme suffix parameter, FacebookUrlSchemeSuffix, in your app’s .plist file.